One thing I've been really working towards is a clean, entity-based design. Over the last decade, I've found that entity-based systems tend to be easier to develop, and are more likely to exhibit emergent gameplay through the interaction of the individual entities.
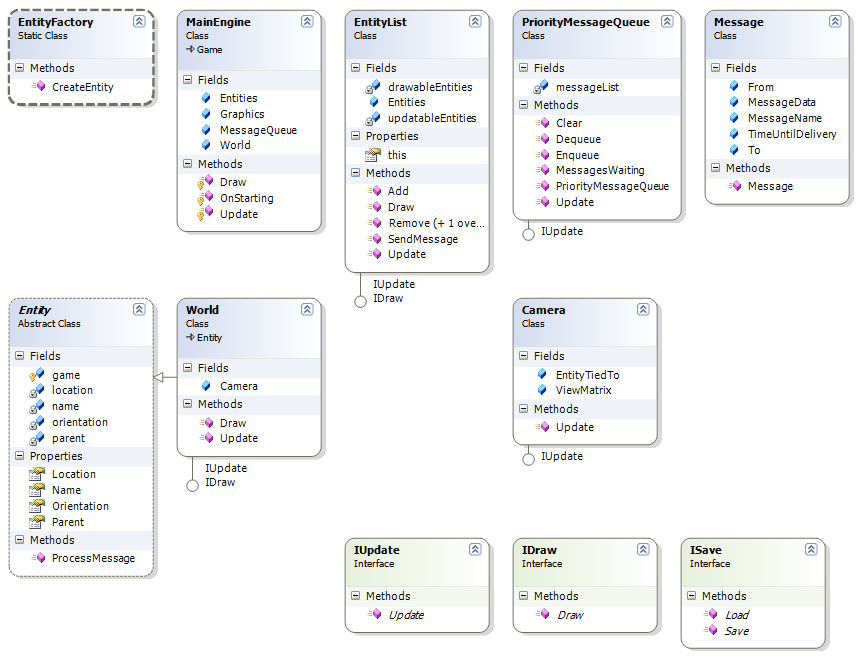
Just a quick rundown before I head out to lunch.
The
EntityFactory
class actually creates the entities based on information passed from the level data. Generally, this is a set of key-value pairs. Based on the value of one of the key-value pairs, it creates and returns the appropriate entity.Each
Entity
has a reference to its parent, with the exception of the World
entity. The reason for this is so we can have related locations and orientations.The
EntityList
class acts as an accelerator for the entity system. When an entity is added, it is checked to see if it implements IUpdate
or IDraw
. If it does, it's added to the appropriate quicklist for the helpers. It also routes messages.The
PriorityMessageQueue
acts as the mailbox for the system. Each Message
object has a time until it is delivered. Handy for objects sending themselves messages for later, or for staged items. MessagesWaiting
only returns true if there are messages whose time has come.One of the XNA component demonstrations was a Camera object, and I thought it was a really poor choice, because cameras rarely exist on their own. They're always tied to something, either a fixed point in space or a moving entity. The
Camera
object I'm using is always attached to an entity. When the camera is updated, it updates the view matrix based on the entity that it is tied to. If, for whatever reason, the camera is pointed to a null entity, I return an identity matrix.Entities do not have to all be drawn. Entities do not have to be updated, either. Some entities could be helpers, like targets or pathnodes. Other entities could be curve markers (using the nice
Curve
functionality built into the XNA Framework, for example). For a cinematic, you could tie your camera to an object that gets moved along the curve. You could implement a logic system ala "SiN Episodes: Emergence" or "Half-Life 2" using entities as well.Game engines work best when they stay simple. Let the complexity arise out of your content, not your code.
1 comment:
Stumbled across your blog. Great post! I am myself currently trying to engineer an entity-based game engine using XNA/C#. I'm an absolute beginner to programming, so it has been an at times painful learning experience. Even though entity/component based engines seem to be ubiquitous, it's difficult to find any information on the engine architecture for such a system. I guess no one expects a beginner to try :)
Post a Comment